Automatisch komponieren mit Python
Einführung
In diesem Kapitel des Python-Kurses stellen wir Tutorial für Musik-Geprägte vor. Wir benutzen Python um eine Input-Datei zu erstellen für das Musik-Programm Lilypond. Unser Python-Programm wird einen beliebigen Text in Musik-Noten übersetzen, d.h. aus Text automatisch ein Musikstück erzeugen. Zu diesem Zweck wird jedes Zeichen des Alphabets durch unser Programm in ein oder mehrere Noten eines Musik-Stücks gewandelt.Lilypond
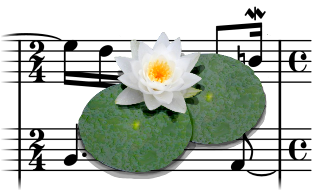
Was ist Lilypond? Die Entwickler von Lilypond definieren es folgendermaßen auf lilypond.org:
"LilyPond is a music engraving program, devoted to producing the highest-quality sheet music possible. It brings the aesthetics of traditionally engraved music to computer printouts."
Weiterhin wird das Ziel wie folgt beschrieben:
"LilyPond came about when two musicians wanted to go beyond the soulless look of computer-printed sheet music. Musicians prefer reading beautiful music, so why couldn't programmers write software to produce elegant printed parts?
The result is a system which frees musicians from the details of layout, allowing them to focus on making music. LilyPond works with them to create publication-quality parts, crafted in the best traditions of classical music engraving."
Ein einfaches Beispiel um mit Lilypond zu beginnen:
\version "2.12.3" { c' e' g' a' }Wenn wir den Code in der Datei simple.ly speichern, können wir Lilypond über die Kommando-Zeile starten:
lilypond simple.lyFolgende Ausgabe wird dabei generiert:
GNU LilyPond 2.12.3 Processing `simple.ly' Parsing... Interpreting music... Preprocessing graphical objects... Finding the ideal number of pages... Fitting music on 1 page... Drawing systems... Layout output to `simple.ps'... Converting to `./simple.pdf'...Das Ergebnis wird in der PDF-Datei "simple.pdf" gespeichert und sieht in etwa so aus:
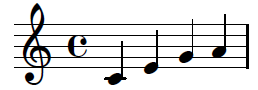
An dieser Stelle möchten wir kein komplettes Lilypond-Tutorial bereitstellen und empfehlen diese Lern-Hilfe.
Python mit Lilypond
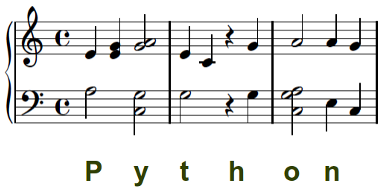
Das Bild auf der rechten Seite stellt die Zeichen-Kette "Python" dar. Wir verwenden eine pentatonische Skala, um sicherzugehen, dass das Ergebnis nicht zu schlecht klingen wird.
Wir implementieren das Mapping mit einem Python-Dictionary:
char2notes = { ' ':("a4 a4 ", "r2 "), 'a':("<c a>2 ", "<e' a'>2 "), 'b':("e2 ", "e'4 <e' g'> "), 'c':("g2 ", "d'4 e' "), 'd':("e2 ", "e'4 a' "), 'e':("<c g>2 ", "a'4 <a' c'> "), 'f':("a2 ", "<g' a'>4 c'' "), 'g':("a2 ", "<g' a'>4 a' "), 'h':("r4 g ", " r4 g' "), 'i':("<c e>2 ", "d'4 g' "), 'j':("a4 a ", "g'4 g' "), 'k':("a2 ", "<g' a'>4 g' "), 'l':("e4 g ", "a'4 a' "), 'm':("c4 e ", "a'4 g' "), 'n':("e4 c ", "a'4 g' "), 'o':("<c a g>2 ", "a'2 "), 'p':("a2 ", "e'4 <e' g'> "), 'q':("a2 ", "a'4 a' "), 'r':("g4 e ", "a'4 a' "), 's':("a2 ", "g'4 a' "), 't':("g2 ", "e'4 c' "), 'u':("<c e g>2 ", "<a' g'>2"), 'v':("e4 e ", "a'4 c' "), 'w':("e4 a ", "a'4 c' "), 'x':("r4 <c d> ", "g' a' "), 'y':("<c g>2 ", "<a' g'>2"), 'z':("<e a>2 ", "g'4 a' "), '\n':("r1 r1 ", "r1 r1 "), ',':("r2 ", "r2"), '.':("<c e a>2 ", "<a c' e'>2") }Die Abbildung eines Strings auf die Noten kann mit einer einfachen Schleife in Python realisiert werden:
txt = "Love one another and you will be happy. It is as simple as that." upper_staff = "" lower_staff = "" for i in txt.lower(): (l,u) = char2notes[i] upper_staff += u lower_staff += lFolgender Code bindet die Strings upper_staff und lower_staff in ein Lilypond-Format ein, welches dann von Lilypond verarbeitet werden kann:
staff = "{\n\\new PianoStaff << \n" staff += " \\new Staff {" + upper_staff + "}\n" staff += " \\new Staff { \clef bass " + lower_staff + "}\n" staff += ">>\n}\n" title = """\header { title = "Love One Another" composer = "Bernd Klein using Python" tagline = "Copyright: Bernd Klein" }""" print title + staffWenn wir den Code unter text_to_musik.py speichern, können wir unseren Klavier-Auszug über folgendes Kommando erstellen:
python text_to_music.py > piano_score.lyWir erhalten eine PDF-Datei mit dem Namen piano_score.pdf.